2022年2月23日
ReactNative初体验flex篇(三)
一般来说,使用flexDirection、alignItems和 justifyContent三个样式属性就已经能满足大多数布局需求。
参考官方文档:https://reactnative.cn/docs/flexbox
备注:1.flex内部可多层嵌套。2.felx总高度固定,子选项超出则顶出去不会影响flex容器变化。3.felx高度不确定时,会选择高度最高的子选择高度撑开。
(3)felx example:
import React, {Component} from "react";
import {StyleSheet, View, Text} from "react-native";
/**
* Created by marno on 2022/2/22.
* Desc:模拟骰子进行布局,算是Flex布局各类属性的实战
*/
export default class FlexDiceTest extends Component {
render() {
return (
<View style={FlexDiceTestStyle.container}>
<Text style={FlexDiceTestStyle.item1}>1</Text>
<Text style={FlexDiceTestStyle.item2}>2</Text>
<Text style={FlexDiceTestStyle.item3}>3</Text>
<Text style={FlexDiceTestStyle.item4}>4</Text>
<Text style={FlexDiceTestStyle.item5}>5</Text>
<Text style={FlexDiceTestStyle.item6}>6</Text>
<Text style={FlexDiceTestStyle.item7}>7</Text>
<Text style={FlexDiceTestStyle.item8}>8</Text>
<Text style={FlexDiceTestStyle.item9}>9</Text>
<Text style={FlexDiceTestStyle.item10}>10</Text>
</View>
)
}
}
const FlexDiceTestStyle = StyleSheet.create({
container: {
backgroundColor: "blue",
height: 450,
width: 300,
//设置主轴(横轴)对齐方式--两端对齐,项目间隔相等。
justifyContent: "space-between",
//规定flex容器是单行或者多行--换行,首行在上。
flexWrap: "wrap",
//布局方向---水平从左到右。
flexDirection: "row",
},
item1: {
color: "#fff",
backgroundColor: "#000",
height: 80,
width: 140,
textAlign: "center",
textAlignVertical: "center",
margin: 4,
},
item2: {
color: "#fff",
backgroundColor: "#000",
height: 80,
width: 140,
textAlign: "center",
textAlignVertical: "center",
margin: 4,
},
item3: {
color: "#fff",
backgroundColor: "#000",
height: 80,
width: 90,
textAlign: "center",
textAlignVertical: "center",
margin: 4,
},
item4: {
color: "#fff",
backgroundColor: "#000",
height: 80,
width: 90,
textAlign: "center",
textAlignVertical: "center",
margin: 4,
},
item5: {
color: "#fff",
backgroundColor: "#000",
height: 80,
width: 90,
textAlign: "center",
textAlignVertical: "center",
margin: 4,
},
item6: {
color: "#fff",
backgroundColor: "#000",
height: 80,
width: 290,
textAlign: "center",
textAlignVertical: "center",
margin: 4,
},
item7: {
color: "#fff",
backgroundColor: "#000",
height: 80,
width: 80,
textAlign: "center",
textAlignVertical: "center",
margin: 4,
},
item8: {
color: "#fff",
backgroundColor: "#000",
height: 80,
width: 80,
textAlign: "center",
textAlignVertical: "center",
margin: 4,
},
item9: {
color: "#fff",
backgroundColor: "#000",
height: 80,
width: 80,
textAlign: "center",
textAlignVertical: "center",
margin: 4,
},
item10: {
color: "#fff",
backgroundColor: "#000",
height: 80,
width: 290,
textAlign: "center",
textAlignVertical: "center",
margin: 4,
}
})
预览:
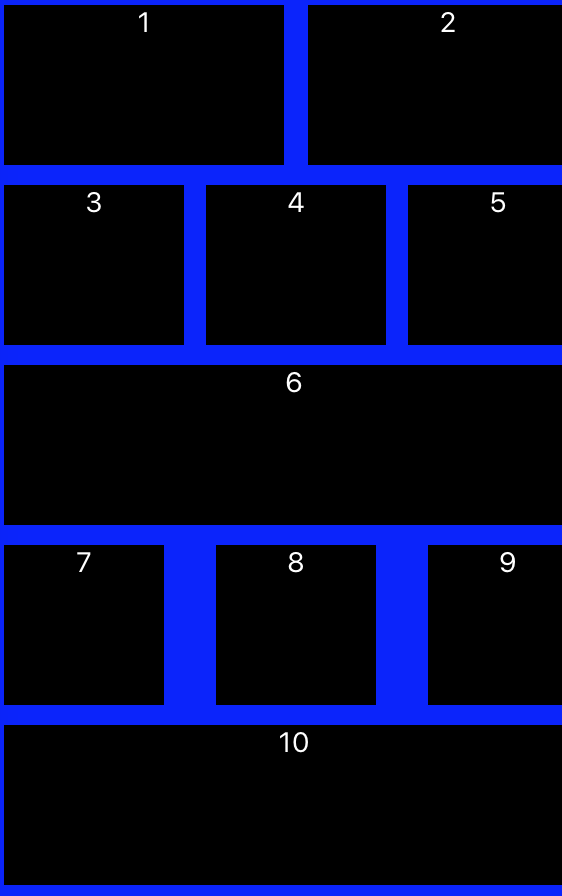